Frame tool allows adding of various scaleable frames to photo or canvas. It works with any photo size or ratio.
var pixie = new Pixie({
onLoad: function() {
var frameTool = pixie.getTool('frame');
frameTool.add('grunge');
}
});
Adding custom frames
Each frame consists of eight parts: Top Left, Top, Top Right, Right, Bottom Right, Bottom, Bottom Left, Left. Each part can have an optional image with either stretch
or repeat
mode. Frame is automatically generated from these eight parts based on canvas size and retina scaling.
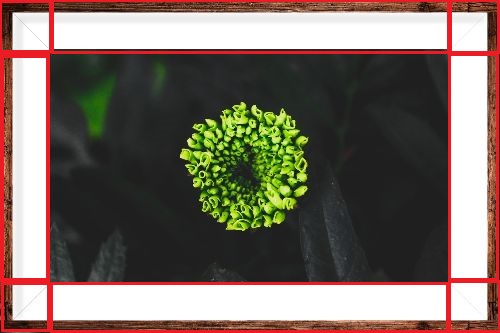
Frame Images
Custom frame images should be placed inside assets/images/frames/{your-frame-name}
folder with matching file names:
- topLeft.png
- top.png
- topRight.png
- left.png
- bottomRight.png
- bottom.png
- bottomLeft.png
- left.png
Frame tool options
replaceDefault
option can be used to hide default pixie frames.
size
option can be used to specify min, max and default frame size in percentages based on current canvas size.
Here is an example code for one of the built in pixie frames:
var pixie = new Pixie({
tools: {
frame: {
replaceDefault: false,
items: [{
name: 'oak',
mode: 'stretch',
size: {
min: 1,
max: 35,
default: 15,
}
}]
}
}
});
Frame tool methods
add
-
Parameters
-
frameName: string
Returns void
-
changeColor
-
Change active "basic" frame color.
Parameters
-
value: string
Returns void
-
getActive
-
Get config of currently active frame.
Returns Frame | null
getAll
-
Get all frame.
Returns Frame[]
getByName
-
Get frame by specified name.
Parameters
-
frameName: string
Returns Frame
-
remove
-
Returns void
resize
-
Resize active frame to specified value.
Parameters
-
value: number
Returns void
-
Add a new frame to canvas.