Pixie currently ships with 18 lightning fast filters that use WebGL, if available, or canvas fallback otherwise and allows you to create custom filters.
var pixie = new Pixie({
onLoad: function() {
var filterTool = pixie.getTool('filter');
filterTool.apply(filterTool.getByName('grayscale'));
}
});
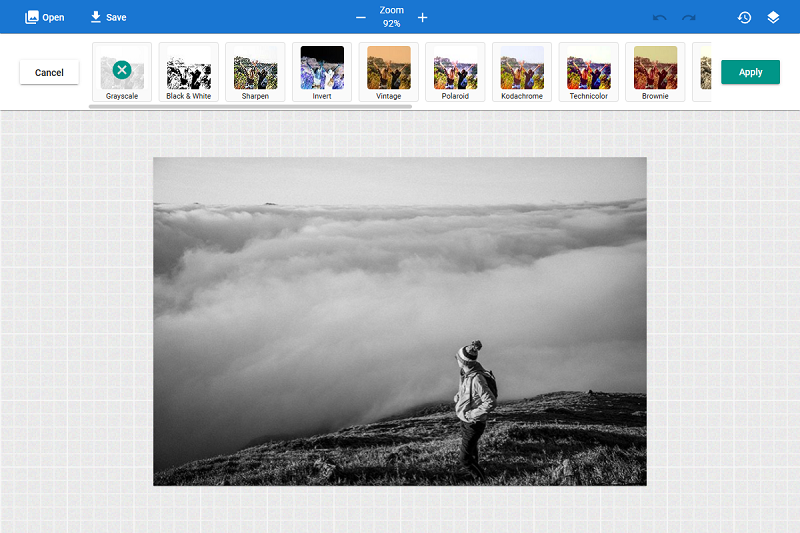
Showing only specific filters
To show only specific filters, specify names of the filters that should be shown using items
option and set replaceDefault
to true
.
var pixie = new Pixie({
tools: {
filter: {
replaceDefault: true,
items: ['brightness', 'noise', 'pixelate'],
}
}
})
Filter tool methods
addCustom
-
Parameters
-
name: string
-
filter: object
-
Optional options: object
Returns void
-
applied
-
Parameters
-
name: string
Returns boolean
-
apply
-
Apply specified filter to canvas.
Parameters
-
filter: Filter
Returns void
-
applyValue
-
Apply specified value to currently active filter.
Parameters
-
filter: Filter
-
optionName: string
-
optionValue: number | string
Returns void
-
create
-
Parameters
-
filter: Filter
Returns IBaseFilter
-
getAll
-
Get all available filters.
Returns Filter[]
getByName
-
Get filter by specified name.
Parameters
-
name: string
Returns Filter
-
remove
-
Parameters
-
filter: Filter
Returns void
-
Add a custom filter to fabric.